In this article, we’ll explore how to create an AWS ECS (Elastic Container Service) cluster using Terraform, a popular infrastructure as code tool.
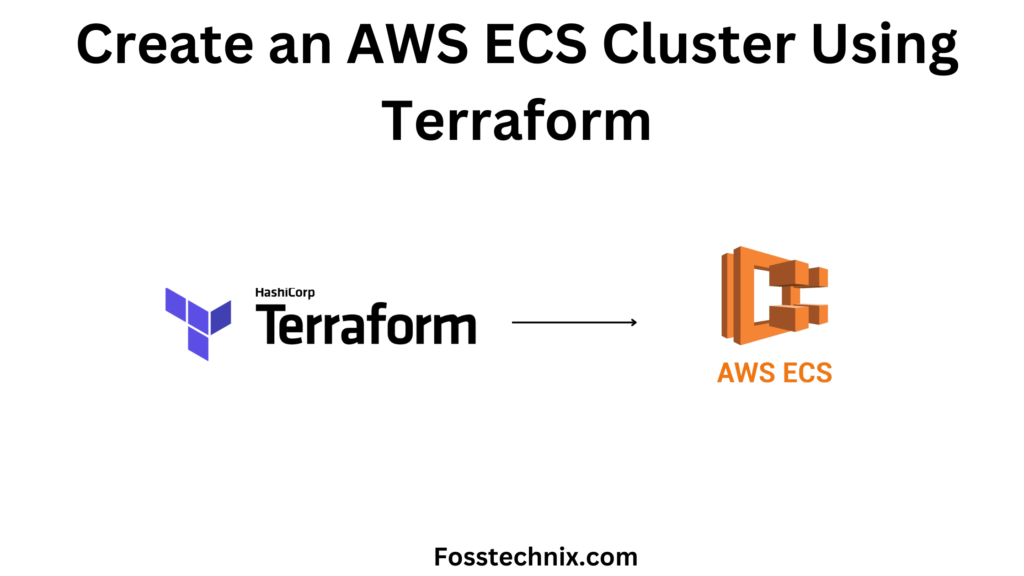
Table of Contents
What is Amazon ECS?
Amazon ECS is a fully managed container orchestration service that allows you to run, stop, and manage Docker containers on a cluster. It takes care of the underlying infrastructure, including the provisioning and scaling of resources, allowing you to focus on your applications. ECS is designed to work seamlessly with other AWS services, providing a comprehensive container management solution.
Architecture of Amazon ECS
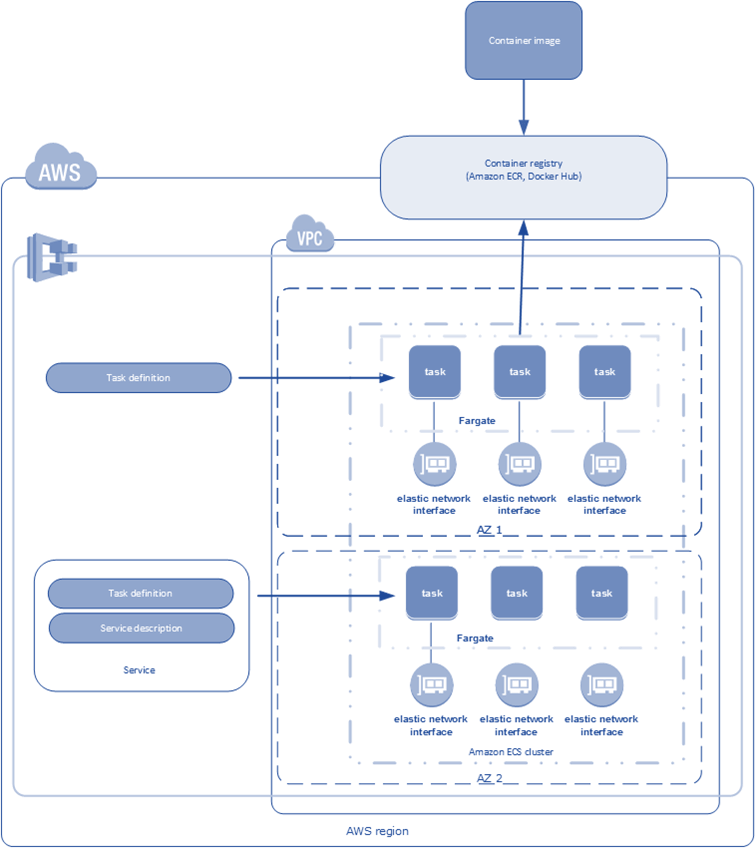
Amazon ECS (Elastic Container Service) architecture comprises several key components that work together to facilitate the deployment and management of containerized applications. Here’s a brief overview:
- ECS Clusters:
- Logical groupings of EC2 instances, AWS Fargate containers, and other resources where containerized applications are deployed.
- Container Instances:
- EC2 instances or AWS Fargate containers within a cluster that host containers and are registered with ECS.
- Task Definitions:
- Blueprints specifying parameters for containers, including Docker image, CPU, memory, and network settings.
- Tasks:
- Instantiation of a task definition, representing the execution of containers on a container instance within the ECS cluster.
- Services:
- Manage continuous running and scaling of tasks, ensuring desired task count, and handling lifecycle management.
- Scheduler:
- ECS component responsible for placing tasks on EC2 instances or AWS Fargate containers, considering resource requirements and availability.
- Amazon ECS Agent:
- Runs on each EC2 instance, facilitating communication with ECS, receiving tasks, and managing container lifecycle.
- Elastic Load Balancing (ELB):
- Distributes incoming traffic across multiple containers within an ECS service for high availability and efficiency.
- Amazon CloudWatch:
- Integrated for monitoring, logging, and tracking metrics to provide insights into ECS task and container performance.
- Amazon ECR (Elastic Container Registry):
- Fully-managed Docker container registry integrated with ECS for secure storage, management, and deployment of container images.
- Container Image:
- A lightweight, standalone, executable package that includes everything needed to run a piece of software. Managed and stored in registries like Amazon ECR.
- Elastic Network Interface (ENI):
- Virtual network interface that allows containers to communicate with the network and other resources in the ECS cluster.
- VPC (Virtual Private Cloud):
- A logically isolated section of the AWS Cloud where ECS clusters and other AWS resources operate, providing control over the network environment.
- AWS Fargate:
- A serverless compute engine for containers that abstracts away infrastructure management, allowing for simplified deployment and operation of containers.
- EC2 Instances:
- Virtual servers in the cloud, forming the foundation of ECS clusters. They host containers when using the EC2 launch type.
Prerequisites
Before you start creating, you’ll need the following:
- an AWS account;
- identity and access management (IAM) credentials and programmatic access;
- AWS credentials that are set up locally with aws configure;
- a code or text editor, like VS Code;
Once you have finished with the prerequisites, it is time to start writing the code to create an ECS cluster.
Steps for Deploying AWS ECS Using Terraform
File Structure:
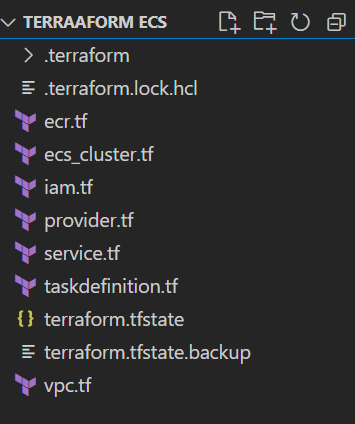
Step#1:Create provider.tf file
The provider.tf file in Terraform is a configuration file that specifies the cloud provider and its corresponding plugin that Terraform will use to manage resources in that provider.
provider.tf
terraform { required_providers { aws = { source = "hashicorp/aws" version = "~> 5.0" } } } provider "aws" { region = "ap-south-1" profile = "default" }

Step#2:Create a virtual private cloud in AWS using Terraform
The next step is to create a virtual private cloud in AWS using the aws_vpc resource.
Let’s name it vpc.tf
vpc.tf
resource "aws_default_vpc" "ecs-vpc" { tags = { Name = "ECS-VPC" } } resource "aws_default_subnet" "ecs_az1" { availability_zone = "ap-south-1a" tags = { Name = "Default subnet for ap-south-1a" } } resource "aws_default_subnet" "ecs_az2" { availability_zone = "ap-south-1b" tags = { Name = "Default subnet for ap-south-1b" } } resource "aws_default_subnet" "ecs_az3" { availability_zone = "ap-south-1c" tags = { Name = "Default subnet for ap-south-1c" } }

Step#3:Create an IAM role by adding iam.tf file
To create an IAM role named “ecsTaskExecutionRole,” you can create an iam.tf
file with the following content:
iam.tf
resource "aws_iam_role" "ecsTaskExecutionRole" { name = "ecsTaskExecutionRole" assume_role_policy = data.aws_iam_policy_document.assume_role_policy.json } data "aws_iam_policy_document" "assume_role_policy" { statement { actions = ["sts:AssumeRole"] principals { type = "Service" identifiers = ["ecs-tasks.amazonaws.com"] } } }
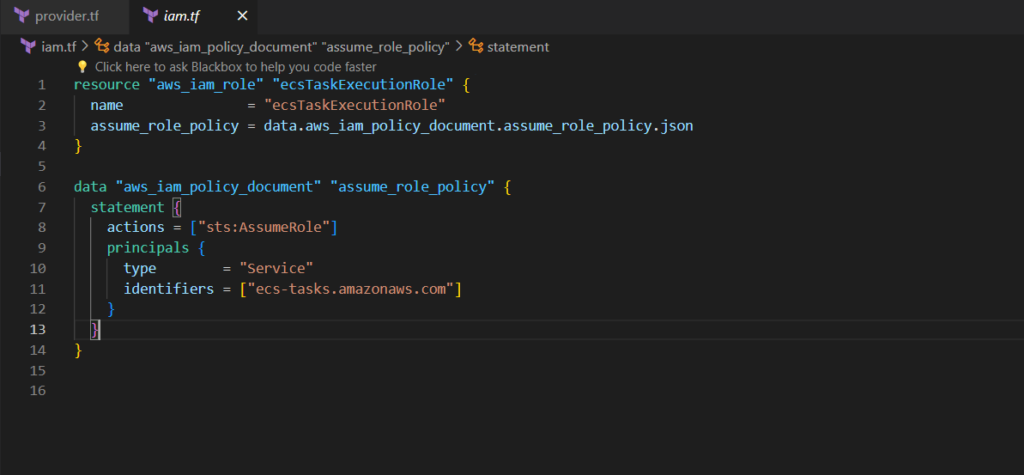
Step#4:Create ecr.tf file
This Terraform file manages AWS ECR repositories for storing container images.
ecr.tf
resource "aws_ecr_repository" "my_first_ecr_repo" { name = "my-first-ecr-repo" tags = { Name="latest_ecr" } }
Save this code in a file named ecr.tf in your Terraform project directory. This file will contain the configuration for managing your AWS ECR repositories.

Step#5:Create taskdefination.tf file
This Terraform file defines an AWS ECS task definition for a containerized application.
taskdefination.tf
resource "aws_ecs_task_definition" "my_first_task" { family = "my-first-task" container_definitions = <<DEFINITION [ { "name": "my-first-task", "image": "${aws_ecr_repository.my_first_ecr_repo.repository_url}", "essential": true, "portMappings": [ { "containerPort": 80, "hostPort": 80 } ], "memory": 512, "cpu": 256, "networkMode": "awsvpc" } ] DEFINITION requires_compatibilities = ["EC2"] network_mode = "awsvpc" memory = 512 execution_role_arn = aws_iam_role.ecsTaskExecutionRole.arn cpu = 256 }
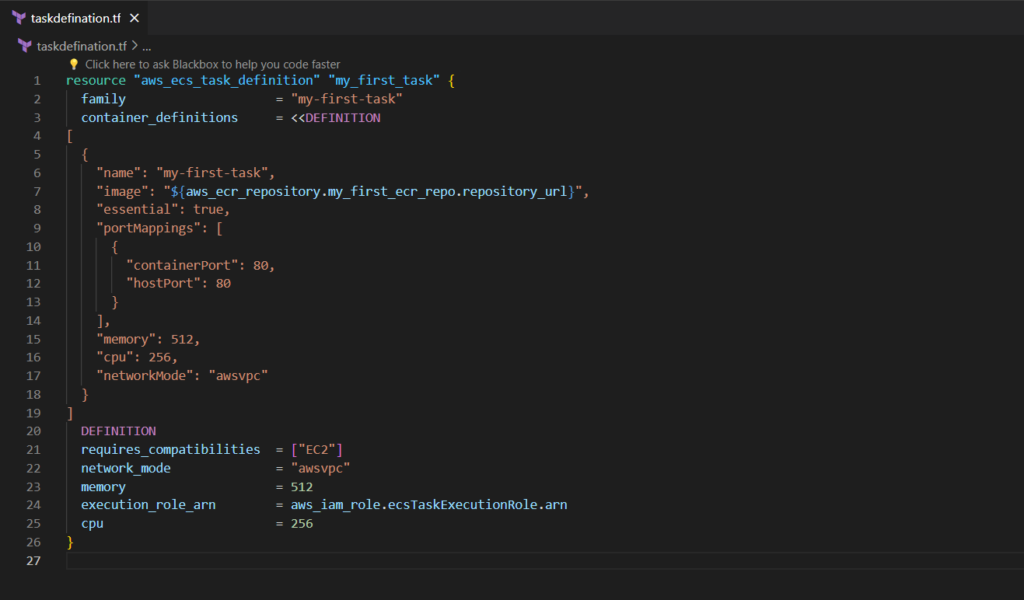
Step#6:Create ecs.tf file
This Terraform file manages AWS ECS resources such as clusters, services, and tasks.
ecs.tf
resource "aws_ecs_cluster" "my_cluster" { name = "my-ecs-cluster" }
Save this code in a file named ecs.tf in your Terraform project directory. This file will contain the configuration for managing your AWS ECS resources.

Step#7:Create service.tf file
This Terraform file manages AWS ECS services for deploying and managing containerized applications.
service.tf
resource "aws_ecs_service" "my_first_services" { name = "gft-test-first-services" cluster = aws_ecs_cluster.my_cluster.id task_definition = aws_ecs_task_definition.my_first_task.arn launch_type = "EC2" scheduling_strategy = "REPLICA" desired_count = 1 network_configuration { subnets = [aws_default_subnet.ecs_az1.id] assign_public_ip = false } }
Save this code in a file named service.tf in your Terraform project directory. This file will contain the configuration for managing your AWS ECS services.

Step#8:Create an Amazon ECS Cluster Using Terraform
- terraform init
The terraform init the command is used to initialize a new or existing Terraform configuration. This command downloads the required provider plugins and sets up the backend for storing state.

- terraform plan
The terraform plan the command is used to create an execution plan for the Terraform configuration. This command shows what resources Terraform will create, modify, or delete when applied.
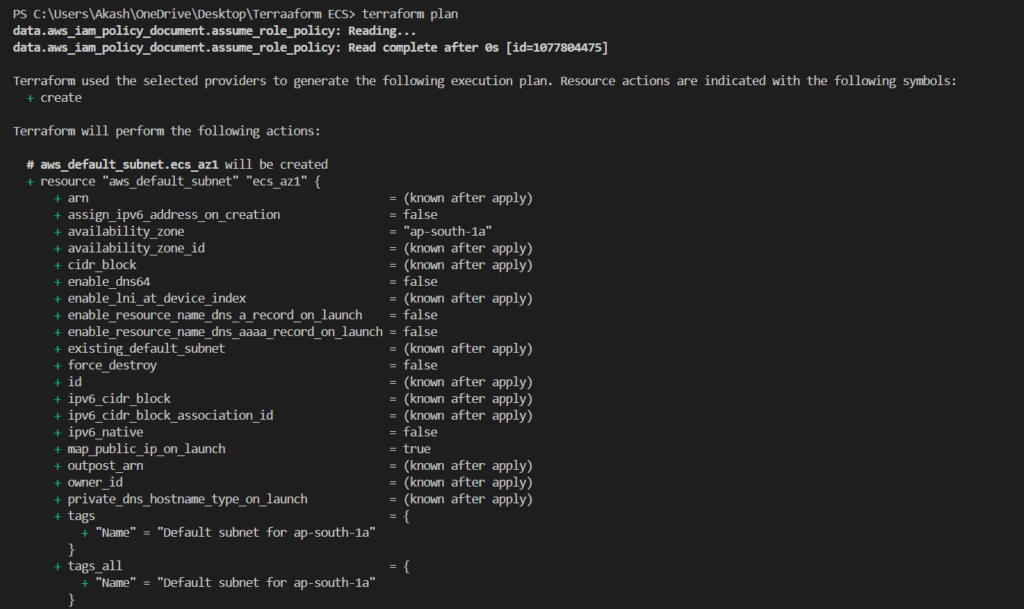
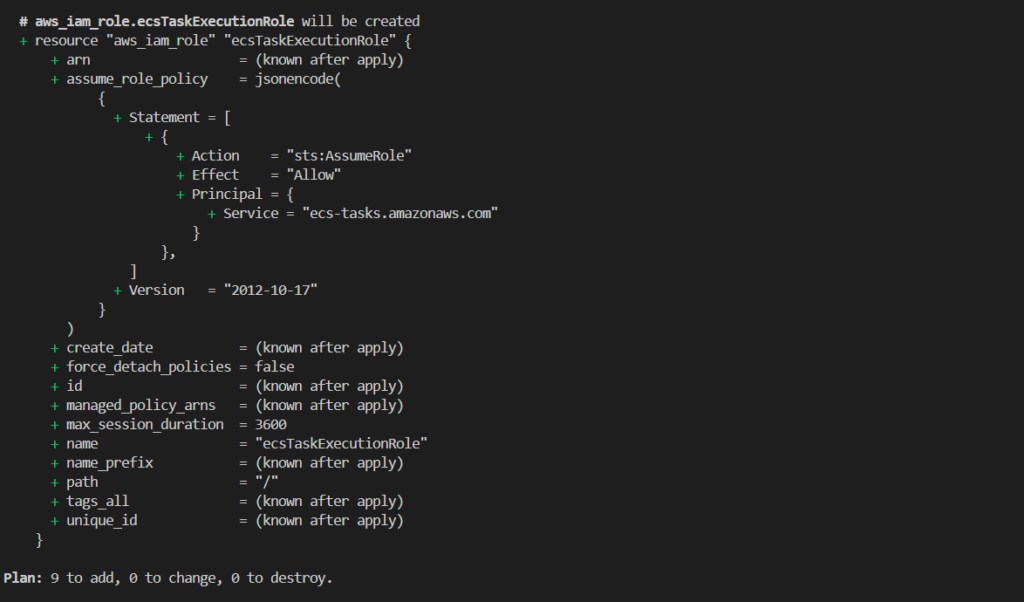
- terraform apply
The terraform apply the command is used to apply the Terraform configuration and create or modify resources in the target environment.
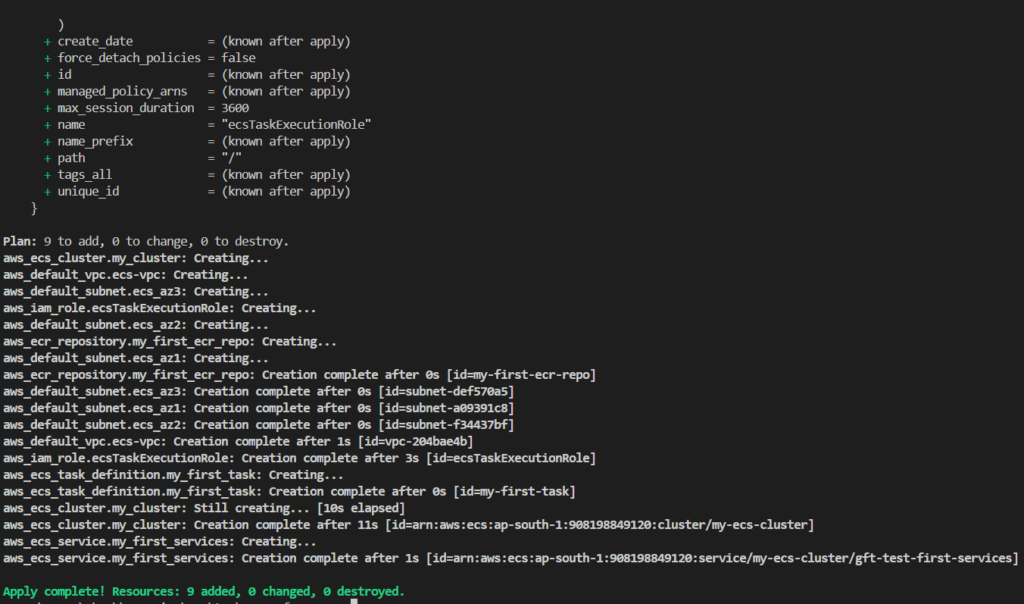
- terraform destroy
All the resources created will be destroy by using this command.
Step#9:Verify Result on AWS Console


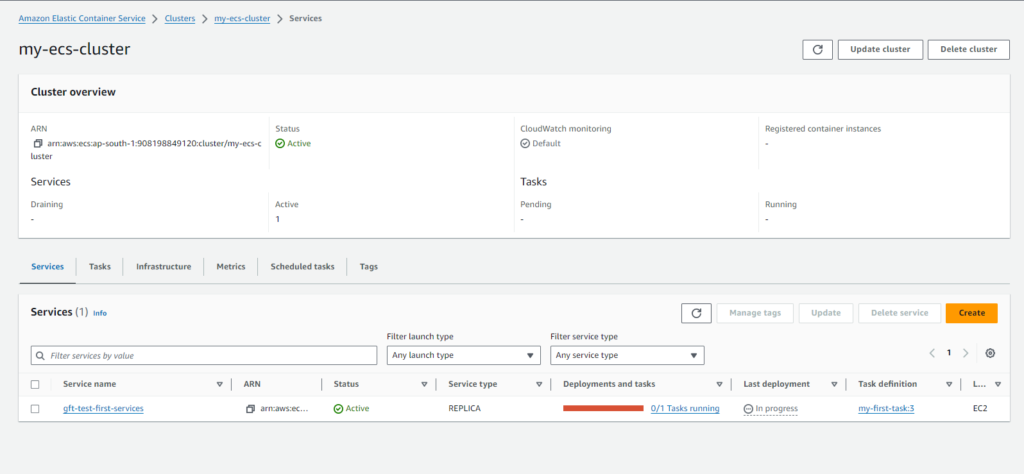
Conclusion
In conclusion, Terraform provides a powerful and efficient way to manage Amazon ECS clusters. By defining infrastructure as code, users can easily create, update, and manage ECS clusters in a repeatable and automated manner. This approach ensures consistency, reduces manual errors, and streamlines the deployment process for containerized applications on AWS ECS.
Reference:-
For reference visit the official website .
Any queries pls contact us @Fosstechnix.com.
Related Articles:
How to Create VPC in AWS using Terraform