In this article, We are going to cover Encapsulation and Polymorphism in Python with Examples, Using + Operator for perform concatenation in Python, Polymorphism with class in Python, Polymorphism with Inheritance in Python.
What is Encapsulation in Python ?
In Python, Encapsulation is a process where we hide the things which is not required by end user. We can restrict access to methods and variables. This prevents data from direct modification which is called encapsulation. We denote private attributes using underscore as the prefix i.e single (_) or double (__).
Example of Encapsulation in Python:
class Mobile:
def __init__(self):
self.__price = 9000
def sell(self):
print("Selling Price: {}".format(self.__price))
def setPrice(self, price):
self.__price = price
c = Mobile()
c.sell()
# using setter function
c.setPrice(10000)
c.sell()
Output:
Selling Price: 9000
Selling Price: 10000
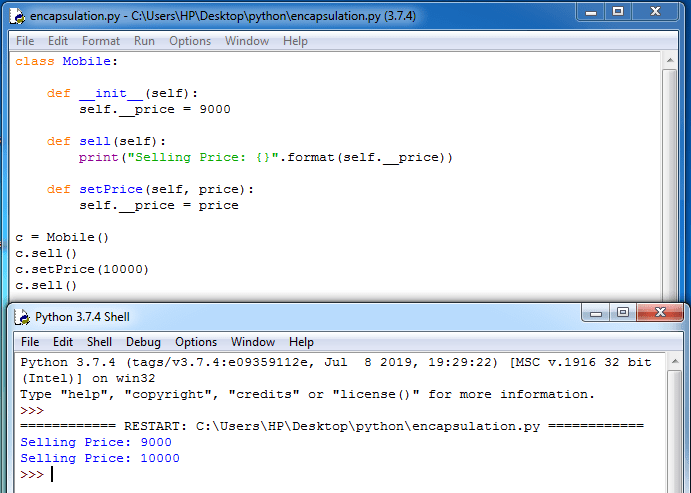
What us Polymorphism In Python ?
It is a process where object behave differently under different circumstances having many forms or single.
Polymorphism is a very important concept in python. It is a single type of entity to represents different types of structure.
Example of polymorphic function in Python:
# len() function used for a string
print(len("FOSSTechNix"))
# len() function used for a list
print(len([22, 33, 44, 55, 66]))
Output:
11
5
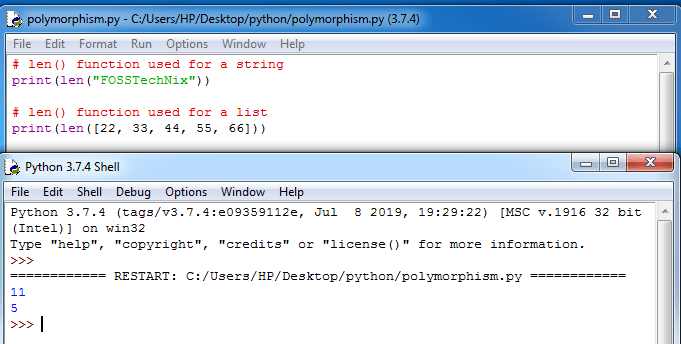
Examples of defined polymorphic function in python
def add(x, y, z = 0):
return x + y+z
def sub(a, b = 0):
return a - b
print(add(2, 3))
print(add(2, 3, 4))
print(sub(7, 3))
print(sub(13, 3))
Output:
5
9
4
10
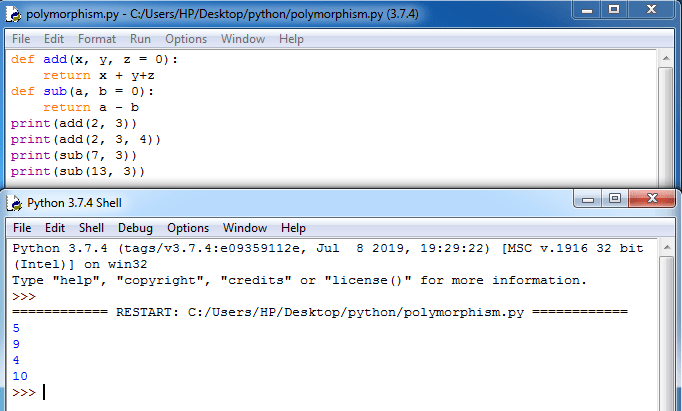
Using + Operator for perform concatenation in Python
Example of concatenation using + operator
str1 = "FOSS"
str2 = "TechNix"
print(str1+" "+str2)
Output:
FOSS TechNix
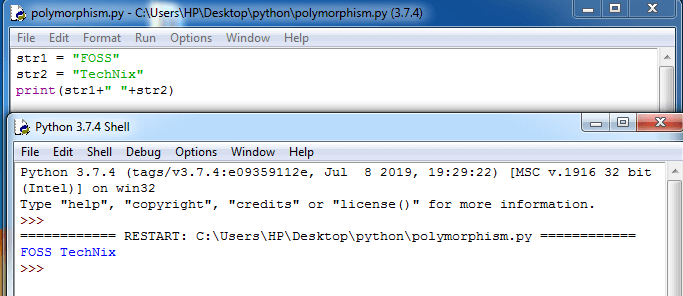
Polymorphism with class in Python:
In python, we can use the concept of polymorphism while creating class methods.
Example of Polymorphism with class in Python:
class Girl:
def __init__(self, name, age):
self.name = name
self.age = age
def info(self):
print(f"I am a girl. My name is {self.name}. I am {self.age} years old.")
def std(self):
print("7th standard")
class Boy:
def __init__(self, name, age):
self.name = name
self.age = age
def info(self):
print(f"I am a boy. My name is {self.name}. I am {self.age} years old.")
def std(self):
print("9th standard")
girl1 = Girl("Lily", 2.5)
boy1 = Boy("Jaxon", 4)
for student in (girl1, boy1):
student.std()
student.info()
student.std()
Output:
7th standard
I am a girl. My name is Lily. I am 2.5 years old.
7th standard
9th standard
I am a boy. My name is Jaxon. I am 4 years old.
9th standard

Polymorphism with Inheritance in Python:
In python, Polymorphism defines methods in the child class that have the same name as the methods in the parent class. Usually child class inherits the method from the parent class.
This process of re-implementing a method in the child class is known as Method Overriding.
Example of Polymorphism with Inheritance in Python:
class Programming:
def intro(self):
print("There are different types of Programming Languages")
def info(self):
print("Some languages difficult to understand and some not")
class python(Programming):
def info(self):
print("Python is programming language")
class java(Programming):
def info(self):
print("Java is programming language")
obj_programming = Programming()
obj_python = python()
obj_java = java()
obj_programming.intro()
obj_programming.info()
obj_python.intro()
obj_python.info()
obj_java.intro()
obj_java.info()
Output:
There are different types of Programming Languages
Some languages difficult to understand and some not
There are different types of Programming Languages
Python is programming language
There are different types of Programming Languages
Java is programming language
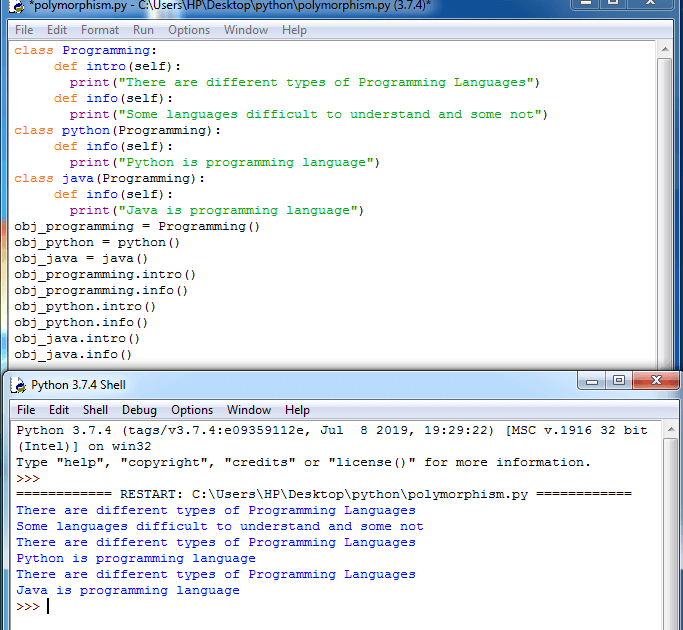
We have covered Encapsulation and polymorphism in python.
Conclusion:
In this article, we have covered Encapsulation and polymorphism in python, Using + Operator for perform concatenation in Python, Polymorphism with class in Python, Polymorphism with Inheritance in Python.
Related Articles:
Functions in Python with Examples
Loops in Python 3 with Examples
7 Python Operators with Examples
Python Introduction for Programmers [Part 1]
6 Python Conditional Statements with Examples
Inheritance in python with examples
Reference: