In this article, Integrate OpenTelemetry for PHP Application with Zero-Code Instrumentation | we will set up OpenTelemetry in a PHP application using the Slim framework with zero-code instrumentation. OpenTelemetry is a robust framework that provides observability for applications by collecting and exporting telemetry data. Zero-code instrumentation means minimal changes to your existing codebase while still gaining valuable insights into application performance.
Table of Contents
Prerequisites
Before you start, ensure you have the following installed on your system.
- AWS Account with Ubuntu 24.04 LTS EC2 Instance.
- PHP, PECL, composer installed.
Step #1:Set Up Ubuntu EC2 Instance
First update the package list.
sudo apt update

Installs the command-line interface for PHP 8.3.
sudo apt install php8.3-cli

Verify the installation.
php -v

Install PECL to install tools needed for PHP extension development.
sudo apt install php-pear php8.3-dev

Download and install Composer. Composer is a dependency manager for PHP that simplifies managing libraries.
curl -sS https://getcomposer.org/installer | php

Move the Composer binary to a directory in /usr/local/bin/, making it accessible globally.
sudo mv composer.phar /usr/local/bin/composer

Checks the version of Composer to verify the installation.
composer -v
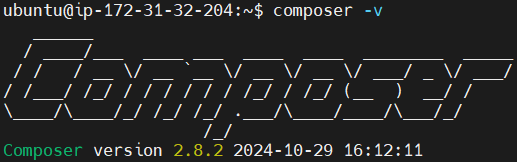
Install Build Tools which are required for building PECL extensions.
sudo apt-get install gcc make autoconf

Step #2:Create Your PHP Project with Slim Framework
Create a new project directory.
mkdir opentelemetry-php-example

Navigate to the directory.
cd opentelemetry-php-example

Initialize a new Composer project, specifying Slim as a dependency.
composer init --no-interaction --require slim/slim:"^4" --require slim/psr7:"^1"

Install the dependencies defined in composer.json using following command.
composer update

Step #3:Create the Application File
Create an index.php file that contains a simple Slim application.
nano index.php

add the following code into it.
<?php
use Psr\Http\Message\ResponseInterface as Response;
use Psr\Http\Message\ServerRequestInterface as Request;
use Slim\Factory\AppFactory;
require __DIR__ . '/vendor/autoload.php';
$app = AppFactory::create();
$app->get('/rolldice', function (Request $request, Response $response) {
$result = random_int(1,6);
$response->getBody()->write(strval($result));
return $response;
});
$app->run();
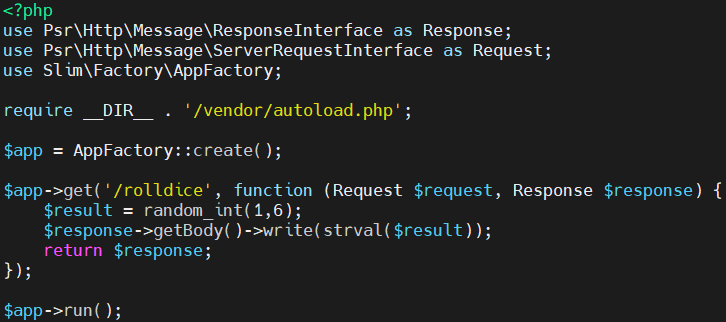
Explanation of the code:
- Imports and Autoloading:
- This imports necessary classes and loads dependencies installed with Composer.
- Create Application:
- Initializes a new Slim application instance. The
$app
variable is now the application object, which will manage routes and handle HTTP requests.
- Initializes a new Slim application instance. The
- Define the
/rolldice
Route:- Defines a route (
/rolldice
) that generates a random integer between 1 and 6, writes it to the response, and sends it back to the client.
- Defines a route (
- Run the Application:
- Starts the application and listens for incoming requests.
Now, start the built-in PHP server to test your application using following command.
php -S 0.0.0.0:8080

Open http://<Public-IP-Address>:8080/rolldice in your browser. You should see a random number between 1 and 6.

Step #4:Integrate OpenTelemetry for PHP Application with Zero-Code Instrumentation
Updates the PECL channel to get the latest available extensions.
sudo pecl channel-update pecl.php.net

Install OpenTelemetry PHP Extension. Use PECL to install the OpenTelemetry extension.
sudo pecl install opentelemetry

After installing the OpenTelemetry extension, you need to enable it in your PHP configuration (php.ini).
sudo nano /etc/php/8.3/cli/php.ini

add the following code into it.
[opentelemetry]
extension=opentelemetry.so

Confirm the extension is installed and enabled
php --ri opentelemetry

Add required packages for automatic instrumentation.
composer config allow-plugins.php-http/discovery false
composer require open-telemetry/sdk open-telemetry/opentelemetry-auto-slim

Step #5:Start the PHP Server with OpenTelemetry
Start the application with the OpenTelemetry environment variables for trace output to the console
env OTEL_PHP_AUTOLOAD_ENABLED=true \
OTEL_TRACES_EXPORTER=console \
OTEL_METRICS_EXPORTER=none \
OTEL_LOGS_EXPORTER=none \
php -S 0.0.0.0:8080

Open http://<Public-IP-Address>:8080/rolldice in your browser and reload.

After you access the application, the console will output trace information for your request, confirming that OpenTelemetry is capturing telemetry data.

Conclusion:
In conclusion, today we have successfully integrated OpenTelemetry in a PHP application using the Slim framework with zero-code instrumentation. This setup allows you to monitor your application’s performance without modifying the application code itself. OpenTelemetry provides valuable insights into the behavior of your application, making it easier to troubleshoot and optimize performance.
Related Articles:
How to Build Spring Boot Project Using Gradle
Reference:
Hi Prasad,
I hope you’re doing well today. I found your post on PHP instrumentation with OpenTelemetry incredibly helpful—thank you so much for sharing it!
Would you happen to have an example of sending this data to an OpenTelemetry receiver endpoint instead of just printing it to the console?
thanks
Would you happen to have an example of sending this data to an OpenTelemetry receiver endpoint instead of just printing it to the console?