In this article we are going to cover 4 Types of String in PHP with Examples.
Table of Contents
What is String in PHP?
PHP string is a sequence of characters, for example, ‘S’ is a character and ‘welcome’ is a string. It is used to store and manipulate text. There are four types to specify a string literal in PHP.
- single quote
- double quote
- heredoc syntax
- nowdoc syntax
1.Single-quote strings in PHP:
It is the easiest way to specify string in PHP. We can create a string in PHP by enclosing the text in a single-quote. This type of string does not process special characters inside quotes.
Example of single-quote strings in PHP:
<!DOCTYPE html>
<html>
<body>
<?php
$str = 'Welcome to FOSS TechNix';
echo $str;
?>
</body>
</html>
Output:
Welcome to FOSS TechNix
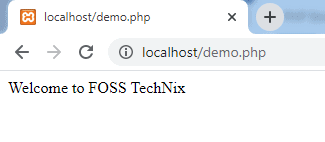
Another Example of single-quote string:
<!DOCTYPE html>
<html>
<body>
<?php
$str = 'FOSS TechNix';
echo 'Welcome $str';
?>
</body>
</html>
Output:
Welcome $str

2.double-quote strings in PHP:
In PHP, double-quote strings in PHP are capable of processing special characters.
Example of double-quote strings in PHP:
<!DOCTYPE html>
<html>
<body>
<?php
$str = "Welcome to FOSS TechNix";
echo $str;
?>
</body>
</html>
Output:
Welcome to FOSS TechNix
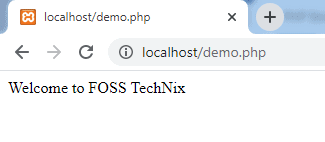
Another Example of double-quote strings in PHP:
<!DOCTYPE html>
<html>
<body>
<?php
$str = "FOSS TechNix";
echo "Welcome to $str";
?>
</body>
</html>
Output:
Welcome to FOSS TechNix
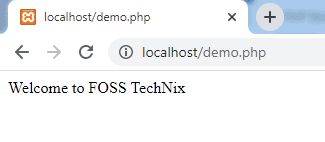
3.Heredoc syntax in PHP:
The Heredoc syntax (<<<) is a another way to delimit PHP strings. In Heredoc syntax, an identifier is given after the heredoc(<<<) operator, after which any text can be written as a new line is started. To close the quotation, the string follows itself and then again that same identifier is provided. That closing identifier must begin from the new line without any whitespace or tab.
Example of Heredoc syntax in PHP:
<!DOCTYPE html>
<html>
<body>
<?php
$text = <<<Heredoc
Welcome to FOSS TechNix.
Heredoc;
echo $text;
?>
</body>
</html>
Output:
Welcome to FOSS TechNix.
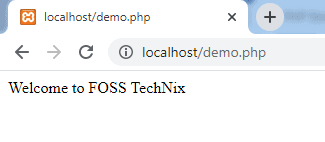
Another Example of Heredoc syntax in PHP:
<!DOCTYPE html>
<html>
<body>
<?php
$test1 = 'FOSS TechNix';
$text = <<<Heredoc
Hello everyone, Welcome to $test1.
Heredoc;
echo $text;
?>
</body>
</html>
Output:
Hello everyone, Welcome to FOSS TechNix.

4.Nowdoc in PHP:
Nowdoc is similar to the heredoc, but in newdoc parsing done in heredoc. It is also identified with three less than symbols <<< followed by an identifier. But in this identifier is enclosed in single-quote e.g. <<<’text’. Newdoc follows same rules as heredoc.
Example of Nowdoc in PHP:
<!DOCTYPE html>
<html>
<body>
<?php
$input = <<<'testNowdoc'
Welcome to FOSS TechNix.
testNowdoc;
echo $input;
// printing string without any variable
echo <<<'Nowdoc'
<br/>
Welcome to FOSS TechNix .
Learning with nowdoc example.
Nowdoc;
?>
</body>
</html>
Output:
Welcome to FOSS TechNix.
Welcome to FOSS TechNix . Learning with nowdoc example.

Built-in String functions in PHP:
In this we will look at some commonly used function to manipulate strings.
Below are some built-in string functions that we use in regular programs.
1.strlen() function in PHP:
The PHP strlen() function is used to find the length of a string. It returns the length of string.
Example of srtlen() function in PHP:
<!DOCTYPE html>
<html>
<body>
<?php
echo strlen("Hello World!");
?>
</body>
</html>
Output:
12

2. strrev() function (Reverse a String):
The PHP strrev() function is used to reverse a string. It returns a reverse string
Example of strrev() function in PHP:
<!DOCTYPE html>
<html>
<body>
<?php
echo strrev("Hello World!");
?>
</body>
</html>
Output:
!dlroW olleH
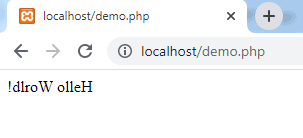
3. str_replace() function (Replace Text within a String) in PHP:
The PHP str_replace() function replace some characters with some
Example of str_replace() function in PHP:
<!DOCTYPE html>
<html>
<body>
<?php
echo str_replace("world", "Shweta", "Hello world!");
?>
</body>
</html>
Output:
Hello Shweta!
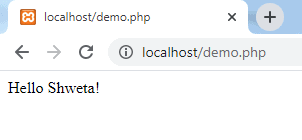
4. strops() function (Search For a Text Within a String) in PHP:
In this function there is two string arguments and if the second string is present in the first one, it will return the starting position of the string otherwise return FALSE.
Example of strops() function in PHP:
<!DOCTYPE html>
<html>
<body>
<?php
echo strpos("Hello FOSS TechNix!", "FOSS"), "<br>";
echo strpos("Hello FOSS TechNix!", "TechNix"), "<br>";
var_dump(strpos("Hello FOSS TechNix!", "for"));
?>
</body>
</html>
Output:
6
11
bool(false)

Conclusion:
We have covered String in PHP with Examples
Related Articles:
- Introduction about PHP [PHP 7]
- Date and Time in PHP with Examples [2 Steps]
- 3 Data Types in PHP with Examples
- Form Validation in PHP with Examples
- 3 Types of Arrays in PHP with Examples
- 4 Types of Loop in PHP with Examples
Reference: