In this article We are going to cover Python Datetime with Examples. In python, there is module datetime to work dates and times.
Example of current datetime in Python:
import datetime
a = datetime.datetime.now()
print(a)
Output:
2020-12-27 17:18:20.230935
Note: The date contains year, month, day, hour, minute, second, and microsecond.
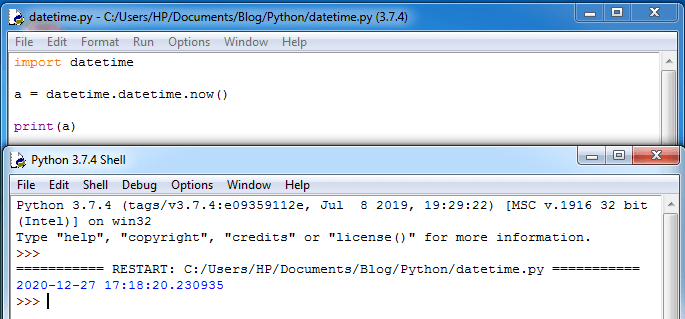
Example of current date in Python:
In this program we use today() method to get today’s date.
import datetime
a = datetime.date.today()
print(a)
Output:
2020-12-27

Example of python datetime:
In this Example return the year and name of weekday.
import datetime
a = datetime.datetime.now()
print(a.year)
print(a.strftime("%A"))
Output:
2020
Sunday
Create Date Object:
The datetime() class requires three parameters to create date: year, month, day.
Example of create date object in python:
import datetime
a = datetime.datetime(2020, 6, 21)
print(a)
Output:
2020-06-21 00:00:00

Example of today’s date, month and year in Python:
from datetime import date
today = date.today()
print("Current year:", today.year)
print("Current month:", today.month)
print("Current day:", today.day)
Output:
Current year: 2020
Current month: 12
Current day: 27

strptime() in Python:
The strptime() method create datetime.
Example of strptime() method in Python:
import datetime
x = datetime.datetime(2020, 6, 21)
print(x.strftime("%d %B, %y"))
Output:
21 June, 20

Example of Current time in Python
import time
x = time.localtime()
time = time.strftime("%H:%M:%S", x)
print(time)
Output:
19:12:33

Digital clock in Python:
In this program we use while loop
Example of digital clock in python:
import time
while True:
l_time = time.localtime()
x = time.strftime("%I:%M:%S %p", l_time)
print(x)
time.sleep(1)
Output:
07:21:16 PM
07:21:17 PM
07:21:18 PM
07:21:19 PM
07:21:20 PM
.
.
.
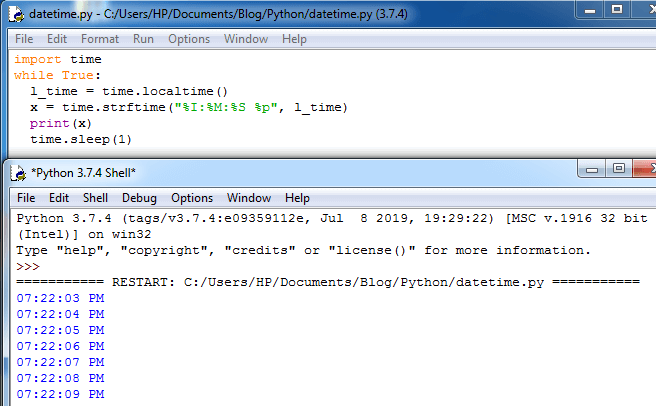
Another Example of digital clock in Python:
import time
while True:
localtime = time.localtime()
result = time.strftime("%I:%M:%S %p", localtime)
print(result, end="", flush=True)
print("\r", end="", flush=True)
time.sleep(1)
Output:
07:28:17 PM07:28:18 PM07:28:19 PM07:28:20 PM07:28:21 PM07:28:22 PM07:28:23 PM07:28:24 PM07:28:25 PM07:28:26 PM07:28:27 PM07:28:28 PM07:28:29 PM07:28:30 PM
07:28:31 PM07:28:32 PM07:28:33 PM07:28:34 PM07:28:35 PM07:28:36 PM

Directive | Description | Example |
%a | Weekday, short version | Mon |
%A | Weekday, full version | Monday |
%d | Day of month 06-21 | 21 |
%w | Weekday as a number 0-6, 0 is Sunday | 3 |
%b | Month name, short version | Jun |
%B | Month name, full version | June |
%m | Month as a number 21-06 | 21 |
%y | Year, short version, without century | 20 |
%Y | Year, full version | 2020 |
%H | Hour 00-23 | 19 |
%I | Hour 00-12 | 07 |
%p | AM/PM | PM |
%M | Minute 00-59 | 48 |
%S | Second 00-59 | 36 |
%f | Microsecond 000000-999999 | 392293 |
%j | Day number of year 001-366 | 365 |
%U | Week number of year, Sunday as the first day of week, 00-53 | 52 |
%W | Week number of year, Monday as the first day of week, 00-53 | 52 |
%c | Local version of date and time | Sun Dec 27 19:54:47 2020 |
%x | Local version of date | 12/27/20 |
%X | Local version of time | 19:56:19 |
Conclusion:
In this article, We have covered Python Datetime with Examples.
Related Articles:
Functions in Python with Examples
Loops in Python 3 with Examples
7 Python Operators with Examples
Python Introduction for Programmers [Part 1]
6 Python Conditional Statements with Examples
Inheritance in python with examples
Encapsulation and Polymorphism in Python with Examples
Python Exception Handling with Examples
Reference: